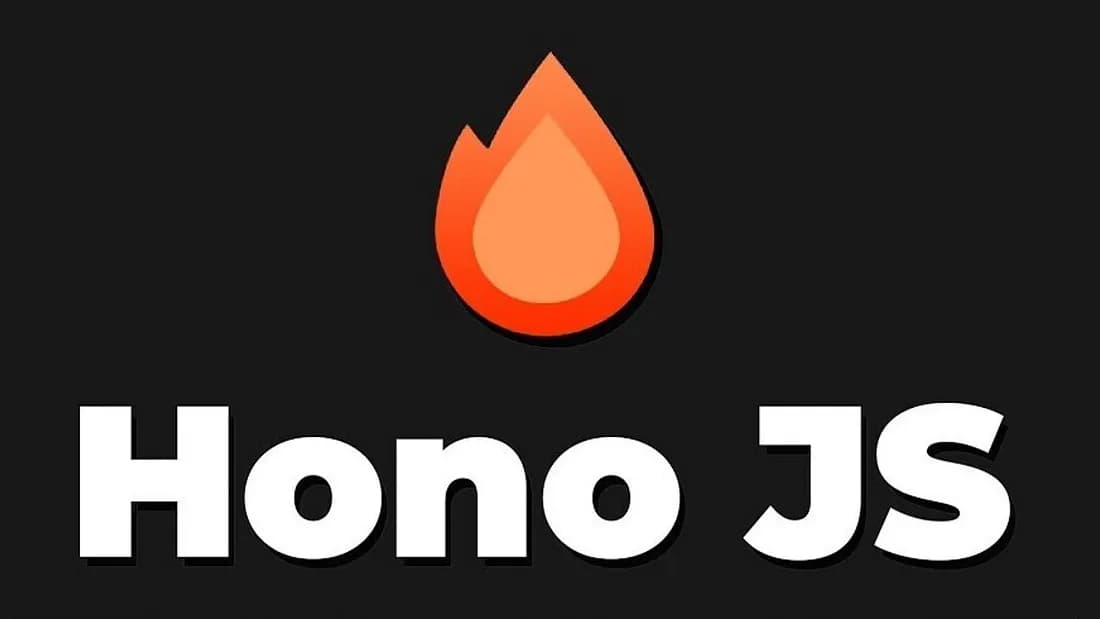
How to Use Bun with Hono - A Lightweight Web Framework
In this guide, you'll learn how to use Hono, a fast, minimal, and modern web framework for building APIs, with Bun, an all-in-one JavaScript runtime like Node.js but faster and more efficient.
We'll go through setting up a Hono project using Bun, creating endpoints, and understanding how to deploy or develop your app effectively.
Why Hono and Bun?
- Bun is a blazing fast JavaScript runtime, bundler, transpiler, and package manager — all in one.
- Hono is an ultra-fast web framework that runs on various JavaScript runtimes including Bun, Cloudflare Workers, and more.
Combining Bun and Hono means you get the benefits of lightning-fast builds and executions along with a modern and minimal web framework.
Prerequisites
Before starting, make sure you have the following installed:
- Bun: If not installed, you can install it using:
curl -fsSL https://bun.sh/install | bash
Click to Copy
After installation, verify it:
bun -v
Click to Copy
Step 1: Initialize the Bun Project
First, create a new Bun project using:
bun init
Click to Copy
Follow the prompts to name your project, choose TypeScript (recommended), and other configurations.
Step 2: Install Hono
Install the Hono package:
bun add hono
Click to Copy
Step 3: Create the Server
Now let’s create a basic Hono server.
// index.ts import { Hono } from "hono"; const app = new Hono(); app.get("/", (c) => c.text("Hello from Hono and Bun!")); app.get("/json", (c) => c.json({ message: "This is a JSON response", }) ); export default { port: 3000, fetch: app.fetch, };
Click to Copy
Here, we define two routes:
- A root route ("/") that returns plain text.
- A JSON route ("/json") that returns a JSON response.
Step 4: Run the Server
Run the server using:
bun index.ts
Click to Copy
Your app will be live at http://localhost:3000
Step 5: Add More Endpoints
You can easily add more routes using Hono's routing system:
app.get("/hello/:name", (c) => { const name = c.req.param("name"); return c.text(`Hello, ${name}!`); });
Click to Copy
Try accessing: http://localhost:3000/hello/sagar
Step 6: Using Middleware
Hono supports middleware. You can log requests or handle authentication like this:
app.use("*", async (c, next) => { console.log(`${c.req.method} ${c.req.url}`); await next(); });
Click to Copy
Step 7: Project Structure (Optional)
For better scalability, you can organize your project like this:
/src /routes index.ts user.ts app.ts server.ts
Click to Copy
Additional Tips
- Hono works seamlessly with TypeScript.
- You can use environment variables with Bun like Node.js via '.env" files.
- Bun also includes a test runner ("bun test") if you want to write tests.
Final Thoughts
Using Bun with Hono gives you an extremely fast and minimal backend setup that is ideal for modern APIs, microservices, or edge functions. It's perfect for developers who want performance and simplicity without sacrificing developer experience.
Explore more about Hono: https://hono.dev
Explore Bun's documentation: https://bun.sh/docs